Debugging SvelteKit server/client code in Visual Studio Code (or WebStorm)
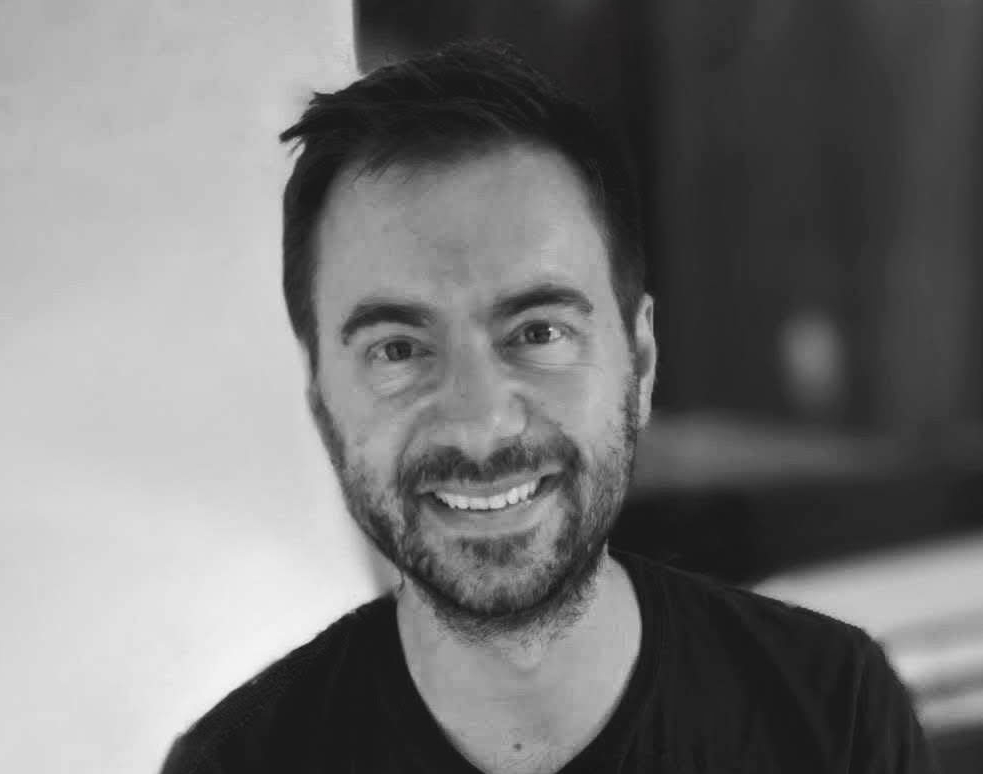
Pro tip: To debug client-side code conveniently in VS Code as well,
- press Ctrl + Shift + P and choose "Debug: JavaScript Debug terminal"
- type your start command (e.g.
pnpm dev
) - Hold down Ctrl when clicking on the URL in the console
Svelte and SvelteKit are amazing, no question about it. One thing that I personally was always longing for, though, is the possibility to debug server-side code in SvelteKit. This is apparently an issue with Vite and sourcemaps (SvelteKit issue, Vite issue).
For this reason, I was thrilled to find out that debugging server code is actually possible - including Typescript support! This works thanks to the vavite library by @cyco130.
Without further ado, here are the simple steps we need to take to get this working:
1. Install the node-loader
from the vavite
library.
pnpm install -D @vavite/node-loader
# or yarn install -D @vavite/node-loader
With this, we can activate Node's experimental ESM loader (only in dev mode), which enables sourcemaps and breakpoints on the server.
2. Use the loader as plugin in your vite.config.js
Add the node-loader
as plugin to your vite config, before the sveltekit()
plugin. In our example, we only do this in development
mode, because this is where we want debugging support.
vite.config.js
import { sveltekit } from '@sveltejs/kit/vite';
import { nodeLoaderPlugin } from '@vavite/node-loader/plugin';
import { defineConfig } from 'vite';
/** @type {import('vite').UserConfig} */
export default defineConfig(({ mode }) => {
let plugins = [sveltekit()];
if (mode === 'development') {
plugins = [nodeLoaderPlugin(), ...plugins];
}
return {
// ... your code ...
plugins
};
});
3. Configure your IDE
Visual Studio Code:
To debug right inside Visual Studio Code, we need to add a simple run configuration. This can be done by adding a launch.json
inside the ./vscode
folder of your application. (Be sure to adapt the port 3000
if you need to):
.vscode/launch.json
{
"version": "0.2.0",
"configurations": [
{
"command": "pnpm vavite-loader vite dev --port 3000",
"name": "Launch SvelteKit server",
"request": "launch",
"type": "node-terminal"
}
]
}
Note that we added our newly added vavite-loader
inside the command
.
WebStorm / IntelliJ Ultimate
For WebStorm (IntelliJ), you have to edit your package.json
directly and change your dev
command in the scripts section to vavite-loader vite dev --port 3000
(or whichever port you prefer). Then, you can create a run configuration (category: npm ) and choose dev
for the run entry. I
f you execute this run configuration in debug mode, you should be able to debug the code right in WebStorm. Pretty neat š.
And that's it š
You should now be able to debug server-side code in Visual Studio Code or WebStorm.
Here's an example, namely a server code file of the official svelte@latest
starter project:
Caveats & Troubleshooting
- The solution is likely to leak memory (source). Arguably, in development mode, this is no big deal, but it's good to have this on your radar.
- Debugging frontend code in VSCode doesn't work (but of course it works in the browser, as usual)
- I think rendering in dev mode is a little bit slower with the additional module loader.
- VSCode might sometimes falsely indicate that a breakpoint can't be hit. However, it will actually work as expected when the code is executed.
- A fix for the underlying Vite issue I mentioned at the beginning has recently been merged into the latest Vite 4.3 beta, so hopefully this workaround is soon no longer needed.
- Generally speaking, with more moving parts, there is a higher risk that something doesn't work, so use at your own risk š
Thanks for reading - Happy coding!
Let's get you some help! Simply paste your URL below: